EncodeQuery.cs
-----------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Security.Cryptography;
using System.IO;
using System.Text;
namespace QueryString
{
public class EncodeQuery
{
private static byte[] key = { };
private static byte[] IV = { 0x12, 0x34, 0x56, 0x78, 0x90, 0xAB, 0xCD, 0xEF };
private static string EncryptionKey = "!#853g`de";
public string Encrypt(string Input)
{
try
{
key = System.Text.Encoding.UTF8.GetBytes(EncryptionKey.Substring(0, 8));
DESCryptoServiceProvider des = new DESCryptoServiceProvider();
Byte[] inputByteArray = Encoding.UTF8.GetBytes(Input);
MemoryStream ms = new MemoryStream();
CryptoStream cs = new CryptoStream(ms, des.CreateEncryptor(key, IV), CryptoStreamMode.Write);
cs.Write(inputByteArray, 0, inputByteArray.Length);
cs.FlushFinalBlock();
return Convert.ToBase64String(ms.ToArray());
}
catch (Exception ex)
{
return "";
}
}
}
}
DecodeQuery.cs
------------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Security.Cryptography;
using System.IO;
using System.Text;
namespace QueryString
{
public class DecodeQuery
{
private static byte[] key = { };
private static byte[] IV = { 0x12, 0x34, 0x56, 0x78, 0x90, 0xAB, 0xCD, 0xEF };
private static string EncryptionKey = "!#853g`de";
public string Decrypt(string Input)
{
Byte[] inputByteArray = new Byte[Input.Length];
try
{
key = System.Text.Encoding.UTF8.GetBytes(EncryptionKey.Substring(0, 8));
DESCryptoServiceProvider des = new DESCryptoServiceProvider();
inputByteArray = Convert.FromBase64String(Input);
MemoryStream ms = new MemoryStream();
CryptoStream cs = new CryptoStream(ms, des.CreateDecryptor(key, IV), CryptoStreamMode.Write);
cs.Write(inputByteArray, 0, inputByteArray.Length);
cs.FlushFinalBlock();
Encoding encoding = Encoding.UTF8;
return encoding.GetString(ms.ToArray());
}
catch (Exception ex)
{
return "";
}
}
}
}
Default.aspx
--------------
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="QueryString._Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div style="height: 138px">
<asp:Button ID="Button1" runat="server" onclick="Button1_Click"
Text="Redirect" />
</div>
</form>
</body>
</html>
Default.aspx.cs
---------------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace QueryString
{
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
EncodeQuery eq = new EncodeQuery();
Response.Redirect("WebForm1.aspx?" + "Name=" + eq.Encrypt("muruga") +"&LastName=" + eq.Encrypt("S"));
}
}
}
WebForm1.aspx
----------------
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebForm1.aspx.cs" Inherits="QueryString.WebForm1" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
</div>
</form>
</body>
</html>
WebForm1.aspx.cs
-------------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace QueryString
{
public partial class WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
DecodeQuery eq = new DecodeQuery();
string str1 = eq.Decrypt(Request.QueryString["Name"]);
string str2 = eq.Decrypt(Request.QueryString["LastName"]);
Response.Write(str1+" ");
Response.Write(str2);
}
}
}
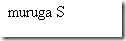