<%@ Page Language="C#" AutoEventWireup="true" CodeFile="DataList2.aspx.cs" Inherits="DUMMY_DataList2" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<table width="900px" align="center">
<tr>
<td>
<asp:DataList ID="DataList1" runat="server">
<HeaderTemplate>
<table width="900px">
<tr style="background-color:Blue;height:30px;color:White;font-size:14px">
<td width="150px">Title</td>
<td width="150px">Message</td>
</tr>
</table>
</HeaderTemplate>
<ItemTemplate>
<table width="900px">
<tr>
<td width="150px"><%# Eval("Title")%></td>
<td width="150px"><%# Eval("Message")%></td>
</tr>
</table>
</ItemTemplate>
</asp:DataList>
</td>
</tr>
<tr>
<td>
<table id="tblPaging" runat="server">
<tr>
<td style="padding-right: 7px" valign="top">
<asp:LinkButton ID="lnkbtnPrevious" runat="server" OnClick="lnkbtnPrevious_Click">Previous</asp:LinkButton>
</td>
<td valign="top">
<asp:DataList ID="dlPaging" runat="server" OnItemCommand="dlPaging_ItemCommand" OnItemDataBound="dlPaging_ItemDataBound"
RepeatDirection="Horizontal">
<ItemTemplate>
<asp:LinkButton ID="lnkbtnPaging" runat="server" CommandArgument='<%# Eval("PageIndex") %>'
CommandName="lnkbtnPaging" Text='<%# Eval("PageText") %>'></asp:LinkButton>
</ItemTemplate>
</asp:DataList>
</td>
<td style="padding-left: 7px" valign="top">
<asp:LinkButton ID="lnkbtnNext" runat="server" OnClick="lnkbtnNext_Click">Next</asp:LinkButton>
</td>
</tr>
</table>
</td>
</tr>
</table>
</form>
</body>
</html>
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
public partial class DUMMY_DataList2 : System.Web.UI.Page
{
PagedDataSource pds = new PagedDataSource();
protected void Page_Load(object sender, EventArgs e)
{
bindDataList();
}
protected void lnkbtnPrevious_Click(object sender, EventArgs e)
{
CurrentPage -= 1;
bindDataList();
}
protected void lnkbtnNext_Click(object sender, EventArgs e)
{
CurrentPage += 1;
bindDataList();
}
protected void dlPaging_ItemCommand(object source, DataListCommandEventArgs e)
{
if (e.CommandName.Equals("lnkbtnPaging"))
{
CurrentPage = Convert.ToInt16(e.CommandArgument.ToString());
bindDataList();
}
}
protected void dlPaging_ItemDataBound(object sender, DataListItemEventArgs e)
{
LinkButton lnkbtnPage = (LinkButton)e.Item.FindControl("lnkbtnPaging");
if (lnkbtnPage.CommandArgument.ToString() == CurrentPage.ToString())
{
lnkbtnPage.Enabled = false;
lnkbtnPage.Font.Bold = true;
}
}
public int CurrentPage
{
get
{
if (this.ViewState["CurrentPage"] == null)
return 0;
else
return Convert.ToInt16(this.ViewState["CurrentPage"].ToString());
}
set
{
this.ViewState["CurrentPage"] = value;
}
}
private void doPaging()
{
DataTable dt = new DataTable();
dt.Columns.Add("PageIndex");
dt.Columns.Add("PageText");
for (int i = 0; i < pds.PageCount; i++)
{
DataRow dr = dt.NewRow();
dr[0] = i;
dr[1] = i + 1;
dt.Rows.Add(dr);
}
dlPaging.DataSource = dt;
dlPaging.DataBind();
}
void bindDataList()
{
string connn = ConfigurationManager.ConnectionStrings["conn"].ConnectionString;
SqlConnection con = new SqlConnection(connn);
con.Open();
string str = "select * from Announcement";
SqlCommand cmd = new SqlCommand(str, con);
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataSet ds = new DataSet();
da.Fill(ds);
DataList1.DataSource = ds;
DataList1.DataBind();
pds.DataSource = ds.Tables[0].DefaultView;
pds.AllowPaging = true;
//to set the paging change the number 3 to your desired value
pds.PageSize = 3;
pds.CurrentPageIndex = CurrentPage;
lnkbtnNext.Enabled = !pds.IsLastPage;
lnkbtnPrevious.Enabled = !pds.IsFirstPage;
DataList1.DataSource = pds;
DataList1.DataBind();
doPaging();
con.Close();
}
}
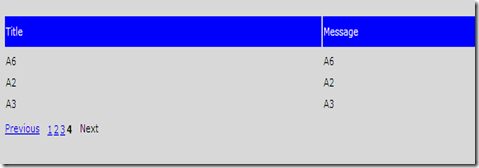